FANUC offers an easy way to command and configure a robot from a PC using their PC Developer's Kit (PCDK).The kit allows a PC to access variables, registers, IO, programs, positions, and alarms on the robot. Most of the help documentation already covers Visual Basic, so I'll explain how to get started with C#.
Installation
First step is to install Visual Studio. Visual Studio Community is free and works perfectly for this application.
After installing Visual Studio, go through the PCDK installer.
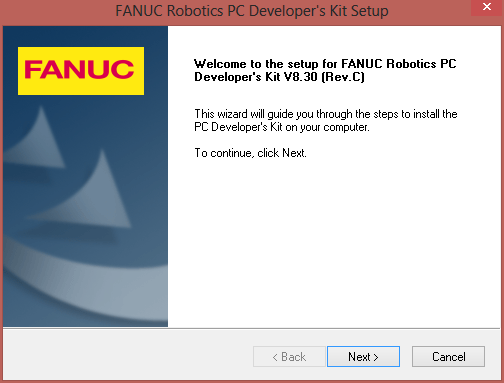
Getting Started with FANUC PCDK
Next, start up Visual Studio and create a new Console Application. I called mine "FanucTest".
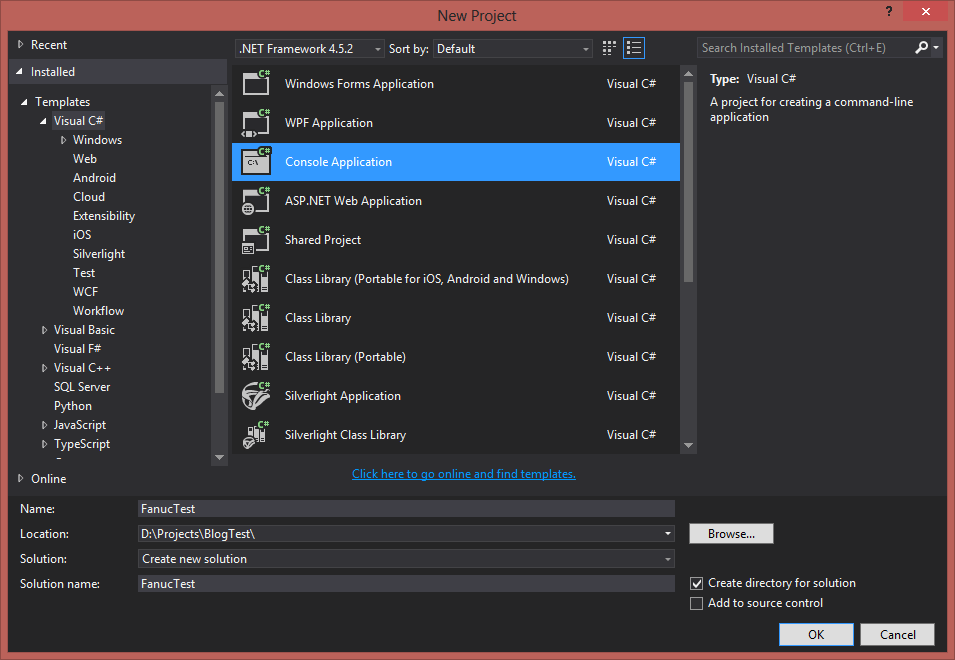
After clicking OK, right click on the "References" in the solution, and click "Add Reference".
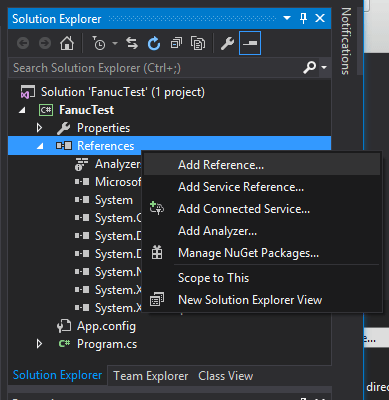
Under COM, find FANUC Robotics Controller Interface. Select it and click OK.
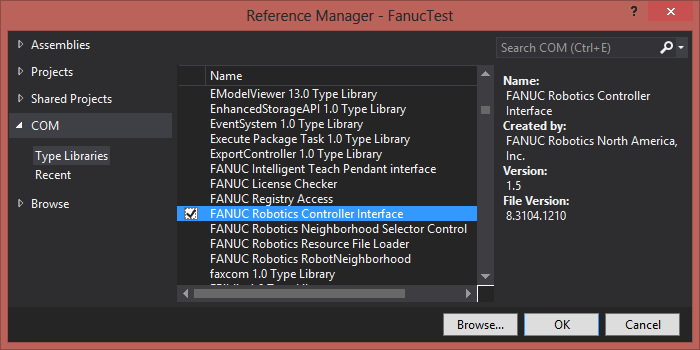
Now to begin writing code!
Connecting to a Robot
Add the following highlighted lines to your code to connect to your robot. If this runs, you've successfully installed PCDK and you're ready to communicate with your robot.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using FRRobot; //uses the robot reference we just added
namespace FanucTest
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Press any key to continue...");
Console.ReadKey();
//Create a robot object
FRCRobot mobjRobot = new FRCRobot();
//Connect to your robot
mobjRobot.Connect("192.168.1.123");
bool connected = mobjRobot.IsConnected;
}
}
}
Updating a Position Register
To finish off this hello world program, add the following lines of code to update a position register. We're accessing position register 1 below and updating the values.
//See help topics "Efficient Access to Positions"
FRCSysPositions sysPositions = mobjRobot.RegPositions;
FRCSysPosition sysPosition = sysPositions[1];
FRCSysGroupPosition sysGroupPosition = sysPosition.Group[1];
FRCXyzWpr xyzWpr = sysGroupPosition.Formats[FRETypeCodeConstants.frXyzWpr];
//Prepare updated X,Y,Z,W,P, and R values of position register 1
xyzWpr.X = 475;
xyzWpr.Y = -275;
xyzWpr.Z = -231;
xyzWpr.W = -176;
xyzWpr.P = 0;
xyzWpr.R = 0;
try
{
//Update position register 1
sysGroupPosition.Update();
}
To learn how to do more with the robot, check out the PCDK help documentation. Depending on your version of Windows, you might need to install another program to view the help documentation.
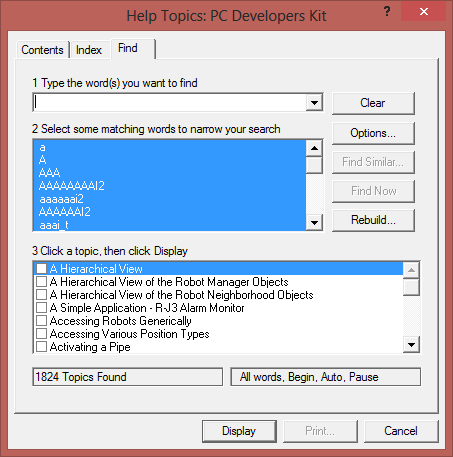
Learn more about DMC's Manufacturing and Automation Intelligence services and contact us today!